For many, the previous post might fall under “news from the margin,” but for me, it’s a highly effective possibility. Now, I’m diving into another tangible topic: JavaScript. Though new to me, JavaScript has quickly captured my full attention. Striving to become a better programmer and advancing as a Web developer, I realized that mastering both Frontend and Backend is essential.
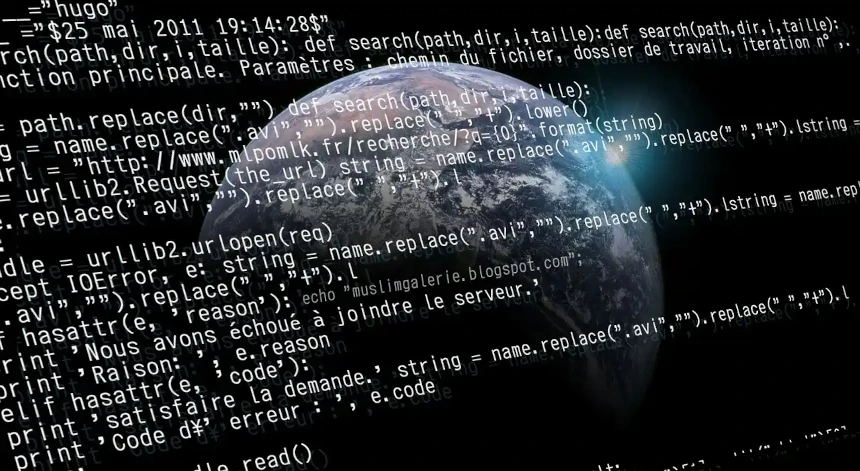
This tutorial covers a popular feature of web pages: smooth scrolling. This effect, commonly embedded in modern web design, is both aesthetically pleasing and professional-looking. The best part? It’s easy to implement. You’ll need some CSS knowledge, as the JavaScript code calls the classes assigned to elements that control this effect. What are these elements? The navigation container (class: .main-navigation) and each individual navigation item (class: .menu-item). Let’s get started!
The code for Smooth Scrolling Effect
if (document.body.classList.contains("home")) { document .querySelector(".main-navigation") .addEventListener("click", function (e) { // Matching strategy if (e.target.parentElement.classList.contains("menu-item")) { e.preventDefault(); const id = e.target.getAttribute("href"); document.querySelector(id).scrollIntoView({ behavior: "smooth" }); } }); }
Script explanation
if (document.body.classList.contains("home"))
With this “if” structure, I’ve ensured the smooth scrolling effect is activated only on the home page, as it contains the “home” CSS class. When the condition is met (the document body includes the “home” class), the smooth scrolling effect will be seamlessly integrated.
document .querySelector(".main-navigation") .addEventListener("click", function (e)`
We focus on the “.main-navigation” class, which is the navigation container of the home page, and we listen to the “click” event on its elements. As soon as a click event occurs, the code from the function is executed.
if (e.target.parentElement.classList.contains("menu-item"))
When a click event occurs within the navigation (as explained in the previous step), we proceed to the next “if” structure to check if the click event happened on one of the navigation elements. If it did, the code within the “if” structure is executed, ensuring smooth scrolling is seamlessly activated.
e.preventDefault();
This line disables the default behavior, and in this case, clicking on the link disables opening of a new page.
const id = e.target.getAttribute("href");
We assign the href link attribute value of the clicked element to the variable id
. This attribute corresponds to one of the sections on the page, with each section linked to a specific menu item.
document.querySelector(id).scrollIntoView({ behavior: "smooth" });
This final line of code enables smooth scrolling to the selected section on the page. By using the scrollIntoView method with an object containing a “behavior” property set to “smooth,” the scrolling effect is seamlessly achieved. The “smooth” value ensures a visually appealing transition, making navigation effortless and engaging.